Wow, what a lot of work to display HTML in an app!
Today’s lesson covered attributed Strings (a string that has associated attributes, such as styles, HTML, etc). Apparently SwiftUI can’t do that yet, so we’re using UIKit to achieve it.
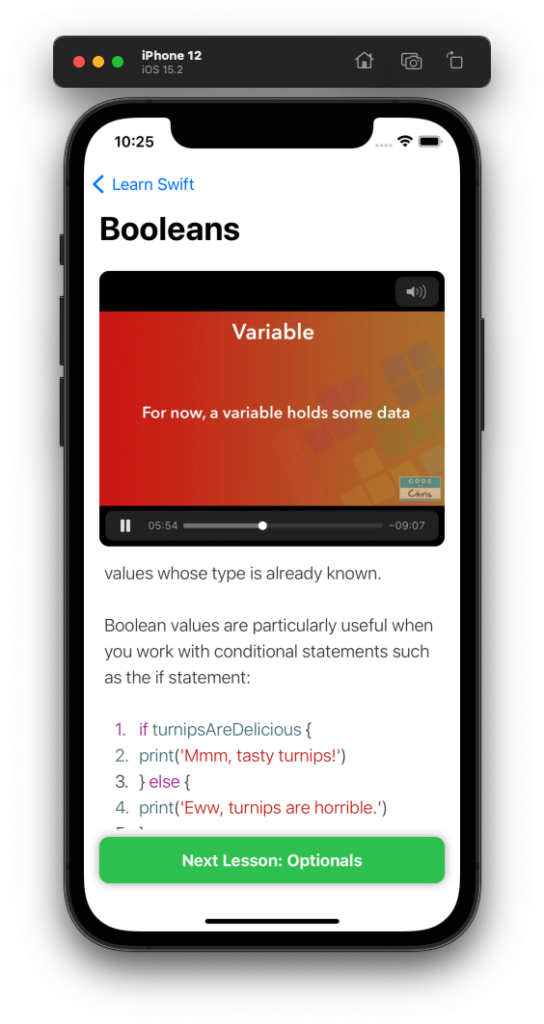
Two methods are required, and the UIViewRepresentable
protocol is used:
struct CodeTextView: UIViewRepresentable {
func makeUIView(context: Context) -> UITextView {
}
func updateUIView(_ textView: UITextView, context: Context) {
}
}
Then we can create a method to add styling to the text:
class ContentModel: ObservableObject {
...
var styleData: Data?
....
private func addStyling(_ htmlString: String) -> NSAttributedString {
var resultString = NSAttributedString()
var data = Data()
if styleData != nil {
data.append(self.styleData!)
}
data.append(Data(htmlString.utf8))
if let attributedString = try? NSAttributedString(data: data, options: [.documentType: NSAttributedString.DocumentType.html], documentAttributes: nil) {
resultString = attributedString
}
return resultString
}
...
}
The result does look pretty good, presuming the data in the JSON file is good HTML/CSS in the first place.
The challenge is to load a webpage using WebKit, which has barely even been mentioned so far. We’ve only just touched on UIKit, and the challenge expects us to already be at the point of mapping that across to WebKit. Think I’ll be looking at the solution, then moving on to the next lesson rather than confuse what we’ve barely learned today with a challenge that spins off into something else. I want to keep my mind focussed.