In Lesson 5 of Module 1 of the CWC+ iOS Database Course, Micah shows us how to use Xcode to add data to our Firestore database.
Having previously set-up Firebase, and ensuring we’ve added GoogleService-Info.plist to our Xcode project, we can use the following commands.
Get access to our Firestore database:
let db = Firestore.firestore()
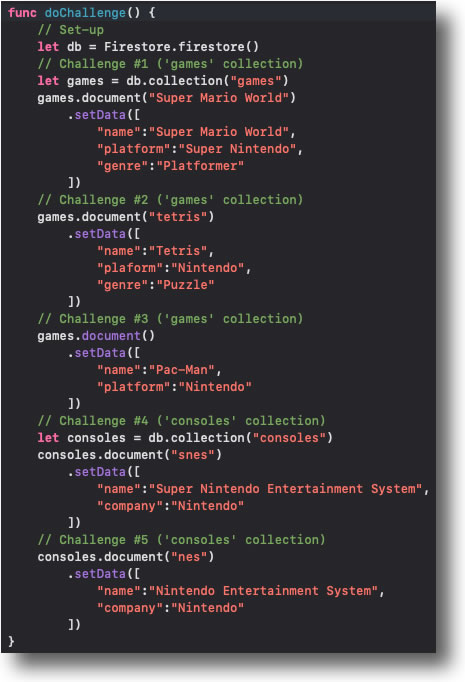
Create a new collection, or get a reference to an existing collection:
let myCollection = db.collection("collection-name")
If the “collection-name” collection doesn’t exist, it will be created.
Create a new document in the collection:
myCollection.document("myDocument")
.setData([
"key1":"value1",
"key2":"value2
])
If we omit the document reference, re: myCollection.document()
, then a unique identifier will be auto-generated. Shorthand for this would be:
let myDocument = myCollection.addDocument(data: ["key1":"value1", "key2":"value2])
So far, so good!