Following on from the previous lesson, this short video teaches us the different ways we can update our Firebase.
let db = Firestore.firestore()
let myDocument = db.collection("collection-name").document("document-name")
We can use the .setData
modifier to, effectively, overwrite all key-value pairs, thus:
myDocument.setData([
"key1":"value1",
"key2":"value2"
])
All key-value pairs will be deleted, and only those listed will be recreated. This has the effect of updating those two keys if they exist, or creating them if they don’t, but also deleting all other key-value pairs.
Alternatively, we can use:
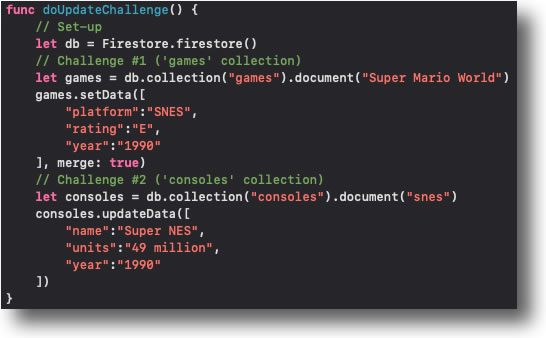
myDocument.setData([
"key1":"value1",
"key2":"value2"
], merge: true)
Set merge: true
to ensure that any fields not listed in setData([:])
are retained and are “merged” with the new data. Any existing key-value pairs lited in setData([;])
will be overwritten (effectively updating them), but no other key-value pair will be affected/deleted.
A better way would be to use the dedicated .updateData
modifier, thus:
myDocument.updateData([
"key1":"value1",
"key2":"value2"
])
This has the same effect as using the .setData
modifier with merge: true
.
All good so far!