Lesson 5 from Module 2 of the CWC+ Design Course gives us an overview of the types of scrollable vertical lists we can use.
Standard ScrollView
ScrollView {
Text("Hello, world!")
.padding()
}
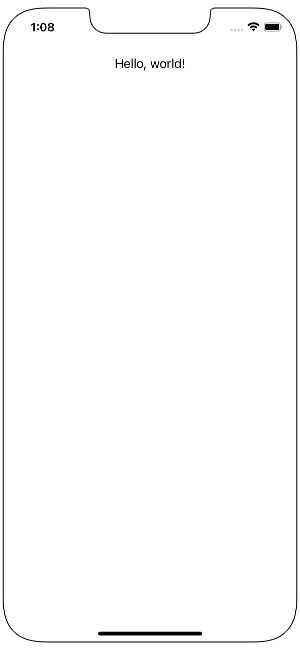
Standard List
var items = ["cat", "dog", "mouse", "bird"]
var body: some View {
List(items, id: \.self) { item in
Text(item)
}
}
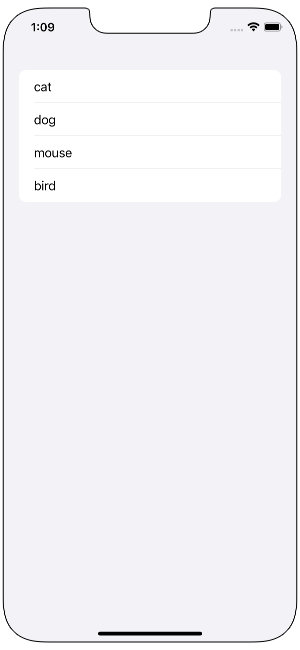
List with ForEach
var items = ["cat", "dog", "mouse", "bird"]
var body: some View {
List {
ForEach(items, id:\.self) { item in
Text(item)
}
}
}
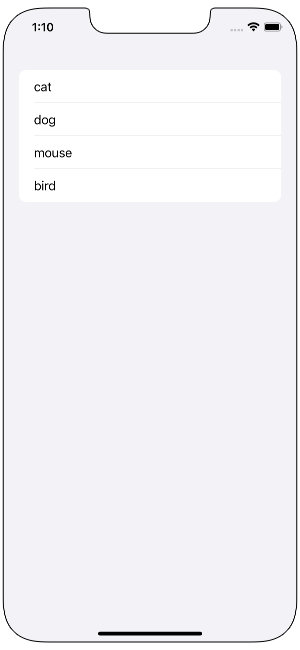
Customisable ScrollView
var items = ["cat", "dog", "mouse", "bird"]
var body: some View {
ScrollView {
VStack{
ForEach(items, id:\.self) { item in
Text(item)
}
}
}
}
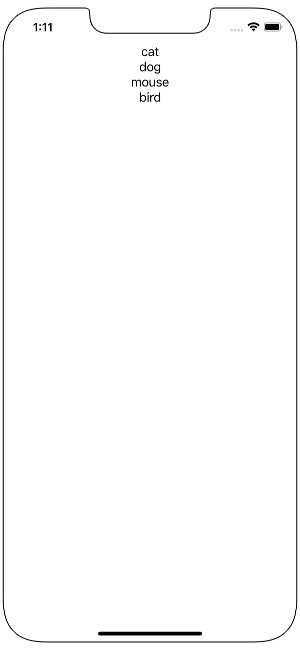
The lesson is couched in the understanding that, at the time the tutorial was filmed, the latest software versions were iOS 14.5 and Xcode 12.5. It is likely that more control / customisation is available with the standard List
element in future versions so the customisable ScrollView
+ VStack
implementation may not be necessary any more.
This tutorial brings home the fact of how quickly computer languages change, and are updated, meaning that learning to code is an ongoing open-ended journey. It’s not something that you can ever class as being “done”.