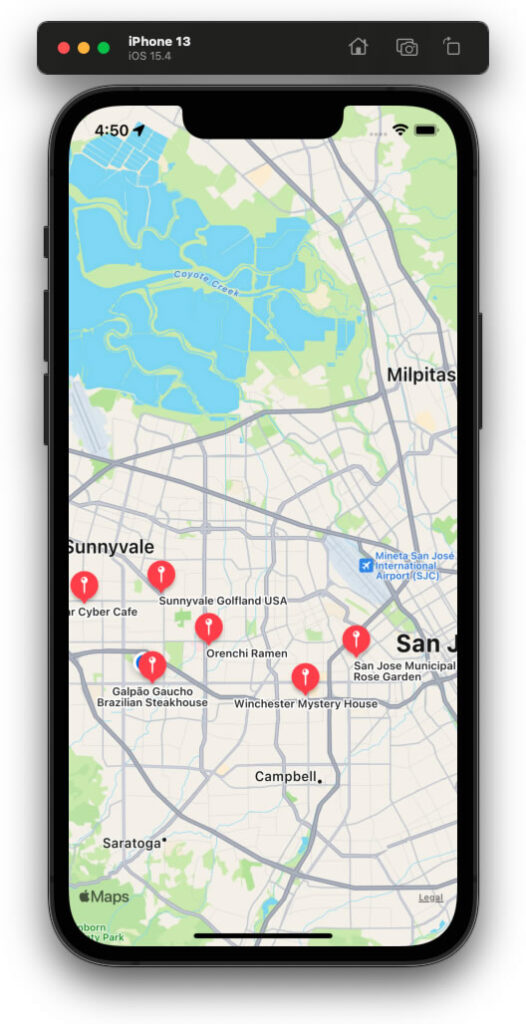
In this lesson, we learned to use the MapView, from UIKit, to show the business landmarks (from the Yelp! data) on the map.
The key components of the code to do this are as follows:
- Method
makeUIView
is required to ensure thestruct
conforms to theUIViewRepresentable
protocol.- It effectively creates the view object and configures its initial state.
- Method
updateUIView
is required to ensure thestruct
conforms to theUIViewRepresentable
protocol.- It updates the state of the specified view with new information from SwiftUI.
- Static method
dismantleUIView
is not marked as required in the SwiftUI documentation, but the lesson teaches us to use it anyway. Not sure what would happen if we didn’t.- It cleans up the presented UIKit view (and coordinator) in anticipation of their removal.
This is our code:
import SwiftUI
import MapKit
struct BusinessMap: UIViewRepresentable {
@EnvironmentObject var model: ContentModel
var locations:[MKPointAnnotation] {
var annotations = [MKPointAnnotation]()
for business in model.restaurants + model.sights {
if let lat = business.coordinates?.latitude, let long = business.coordinates?.longitude {
let a = MKPointAnnotation()
a.coordinate = CLLocationCoordinate2D(latitude: lat, longitude: long)
a.title = business.name ?? ""
annotations.append(a)
}
}
return annotations
}
func makeUIView(context: Context) -> MKMapView {
let mapView = MKMapView()
mapView.showsUserLocation = true
mapView.userTrackingMode = .followWithHeading
return mapView
}
func updateUIView(_ uiView: MKMapView, context: Context) {
uiView.removeAnnotations(uiView.annotations)
uiView.showAnnotations(self.locations, animated: true)
}
static func dismantleUIView(_ uiView: MKMapView, coordinator: ()) {
uiView.removeAnnotations(uiView.annotations)
}
}
The highlighted sections are important. Just leaving it here for future reference.