Okay, so feeling a little brave, I decided to take a step back and allow myself as much time as I needed for this wrap-up challenge. I wanted to see how far I could get using just what I can remember from iOS Foundations up to this point, and what I can locate in previous coding that we’ve done.
Answer: Not a lot!
I thought, at first, to do my old favourite of keeping the URL of the JSON file separate from the parsing script. I never like mixing things like absolute URLs in with the code because you never know when you want to move the file, and trawling through code to find where you’ve inserted it is not a great use of your time. Or, for example, you might want to use the same piece of code for multiple URLs.
It doesn’t need to be separate from the code for this challenge, but I know it’ll be something that will prove useful later in my coding future.
But – boy!, how complicated does this need to be? Wow.
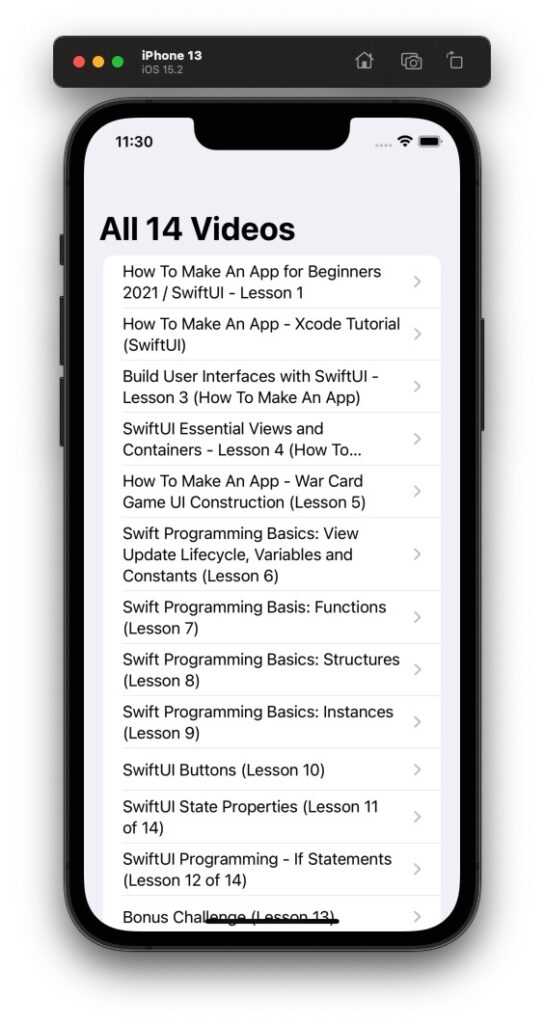
I thought I’d be able to instantiate the class, and then send the URL to the method as a parameter. Nope, not a chance. I could do this back in the days of PHP but, it appears (and I’m only guessing here) that, being view-based, the view “loads” before the URL is recognised and used so the method doesn’t actually have any JSON data to parse. Doesn’t make a lot of sense, but who said coding ever did?
The solution, after doing much head-scratching, is to use multiple views. I’ve changed the code so that the class initialises the URL with a parameter it is sent from the HomeView so that it’s ready when HomeView hands over the view to ContentView. That probably sounds like a whole load of gobbledegook – it certainly does in my head! – but it seems to work. I’m pleased that I found a solution.
I’ve had to rely heavily on the challenge solution to remind me of various aspects that should, by now, be simple. I can only believe that there is so much confusion in my head trying to keep track of how to do the simple things (that are complicated), that I can’t keep track of the things we rarely use. Must be my age.
The problem with learning via video tutorials is that Chris never “makes mistakes” (except those that are not his – like when an update to Xcode/iOS breaks things), so we don’t see how to overcome mistakes when they happen. And they do happen. With alarming frequency. Xcode pretty much demands you code the “right way” and it won’t give you any lattitude for coding in a way that “seems to make sense at the time”. The result? Lots of red Xs and cryptic messages as it frequently, oh so frequently, fails to build or show you a preview.
I can only hope that something, somewhere, is sinking into my head. I can do more than I could do when I started out on this course five weeks ago, and I’m pleased about that more than I can say, but I feel that I should at least have more of the basics down by now,.
The wrap-up challenge, which is already complicated enough, also has an extra challenge (optional) in that the app includes a ‘search bar’ – of which we’ve never even touched on before. The search should filter the results as you type, and I’ve no clue at all how to implement that. This is optional and is not part of the original challenge, but I’m at least going to have a trawl through the solution and try to cram something even more complicated into my head that’s already crammed full of complicated stuff. It’s what I do.
Coding : a word that shares its first two letter with “complicated” and “complex” and “convoluted”.
Addendum:
Okay, so I’ve watched Chris’ “hints” video on how to add the SearchBar. Can anyone say “whooosh!” as that all flew over my head? Twice I’ve tried to watch this, and twice I’ve paused it part way and walked away. Cripes, that’s a LOT to take in!
I vaguely get the notion that we have to use an UIKit SearchBar because SwiftUI doesn’t have one built in. But then Chris races over things like ‘delegate’, ‘coordinator’, ‘textDidChange’, multiple methods, class within struct, and …. wow, I just can’t keep up with him.
At this point I feel that any attempt to add the ‘search bar’ into the Wrap-Up Challenge would be nothing more than a copy & paste affair, taking code from the solution and not knowing what any of it does. That seems pretty pointless, so I’m not going to do that.
I guess it’s time to call Module 5 complete. Not quite the “resounding success” and “congratulations all-around” that I’d envisaged. I can only hope that the more of these “following Chris doing his coding” videos that I follow, the more I might begin to understand. Right now, that ocean still seems just as big as it always was.
Addendum #2:
Never one to give up on something half-finished, I did a bit of searching and discovered that, in actual fact, iOS 15 does have its own searchbar function.
struct ContentView: View {
@ObservedObject var model: DataUtils
@State private var searchText = ""
var body: some View {
if model.jsonDataFile.count > 0 {
NavigationView {
List {
ForEach(searchResults) { data in
NavigationLink(data.title,
destination: VideoScreen(video: data))
}
}
.navigationTitle(Text("All " + String(model.jsonDataFile.count) + " Videos"))
}
.searchable(text: $searchText)
}
else {
ProgressView()
}
}
var searchResults: [DataModel] {
if searchText.isEmpty {
return model.jsonDataFile
} else {
return model.jsonDataFile.filter { $0.title.contains(searchText)}
}
}
}
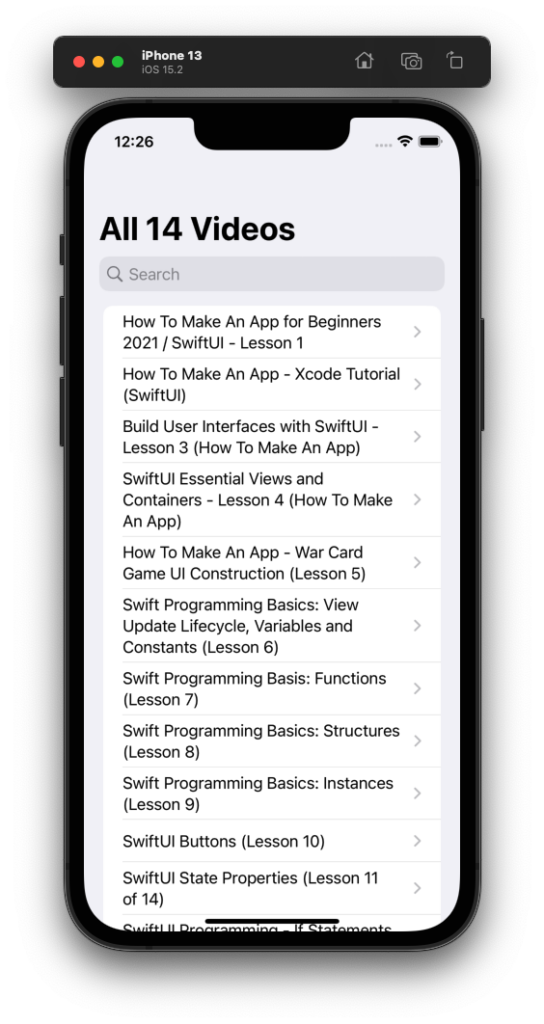
Everything that’s highlighted
belongs to the SearchBar functionality. Without the Search Bar, the ForEach
statement was:
ForEach(model.jsonDataFile) { data in
...
}
Everything else is just added to the code.
I’m glad I didn’t get too bogged down with the previous complicated convoluted kludge to get the SearchBar functionality working. I don’t think I would have had any hair left by now!
Now, at last, finally, I can put Module 5 to bed and move on to Module 6…