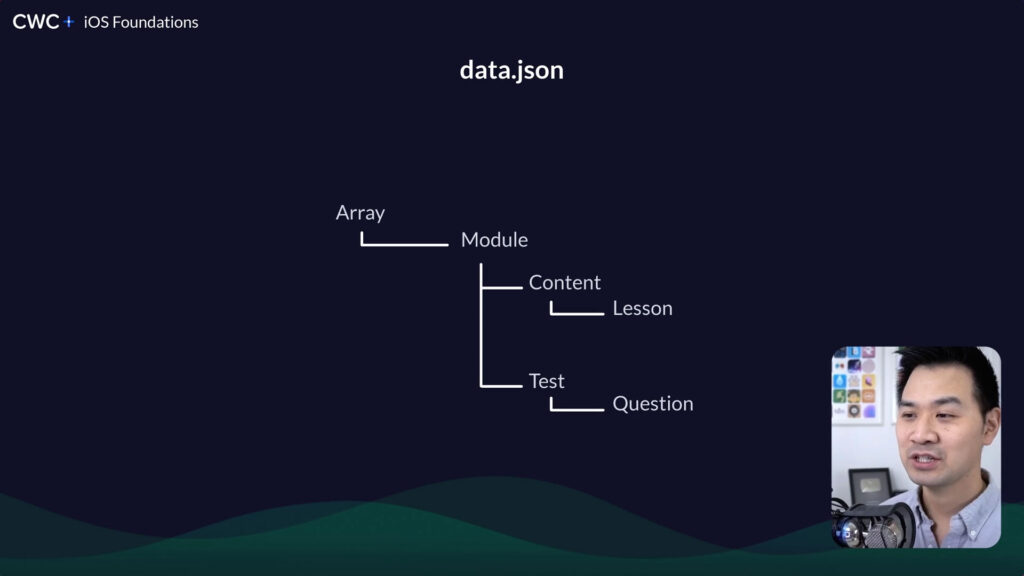
Today’s lesson takes advantage of any talent you may have when it comes to keyboard dexterity. It consists mostly of following Chris through setting up the JSON parsing. Keeping up with his keyboard speed requires above average keyboard dexterity, and even I had to hit ‘pause’ more than once for this.
Refamiliarising ourselves concepts that we’ve used before, Lesson 3 of Module 5 of iOS Foundations takes us through setting up the models required to get the JSON data.
Models are created, thus:
struct Module: Decodable, Identifiable {
// set-up vars
}
Here the models must conform to the Decodable
protocol (because we need to decode the JSON data) and the Identifiable
protocol because we’ll be looping through them using ForEach
or List
.
We’ve also set up the method for parsing the JSON data. A typical method for this, where the JSON data is located in the ‘data.json’ file, is:
class ContentModel: ObservableObject {
@Published var modules = [Module]()
init() {
getLocalData()
}
func getLocalData() {
let jsonUrl = Bundle.main.url(forResource: "data", withExtension: "json")
do {
let jsonData = try Data(contentsOf: jsonUrl!)
let jsonDecoder = JSONDecoder()
let modules = try jsonDecoder.decode([Module].self, from: jsonData)
self.modules = modules
}
catch {
print("Couldn't parse local data")
}
}
}
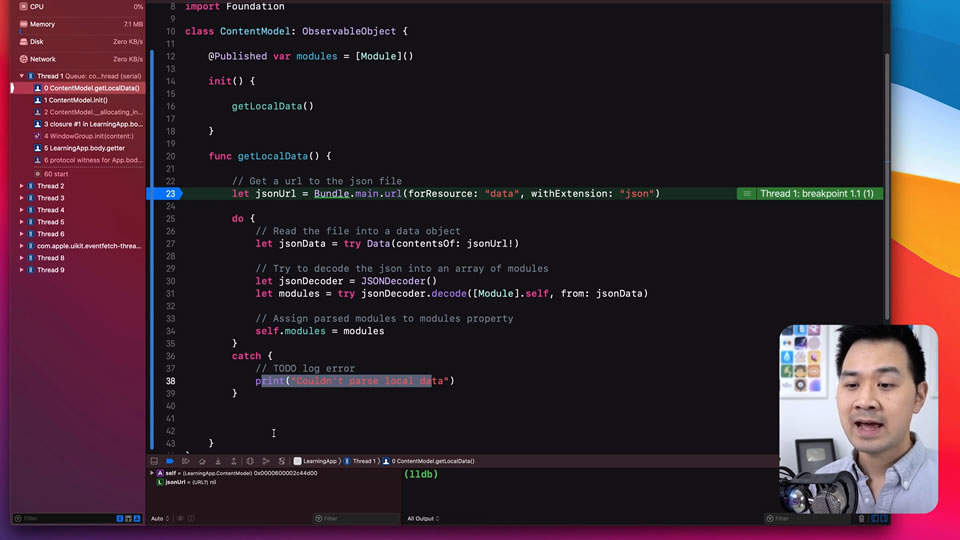
This time around we’ve also looked at bringing another type of data file into the project – HTML data inside an array within the JSON data, and a CSS StyleSheet data file for formatting that HTML later.
We’ve set up a model to parse the stylesheet (which, obviously, doesn’t require JSONDecoder()
because it’s not JSON).
Using the simulator to check that we haven’t made any glaring errors in our rapid typing, and we’re good to go for the next lesson…
More to follow …